Top 5 Problems Google Leaves to Third-Party Vendors for Android Developers
Critical Gaps in Android Development: Where Google Relies on Third-Party Solutions
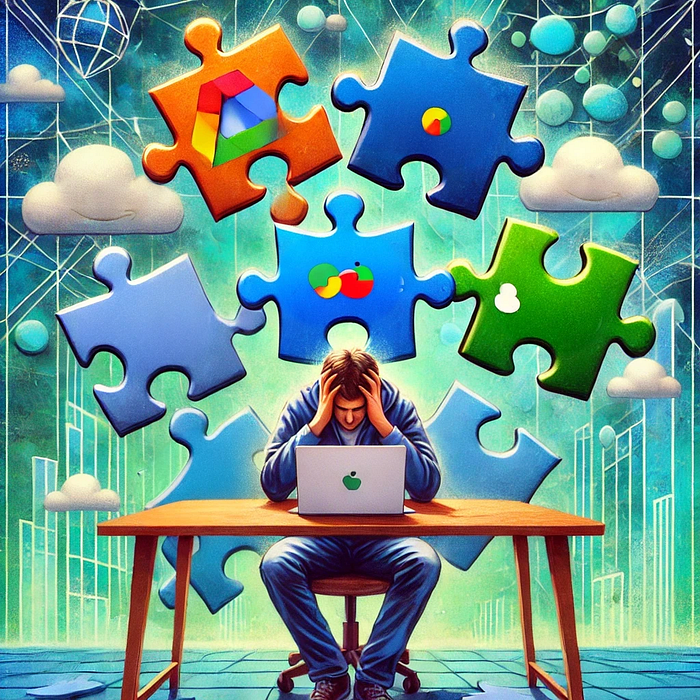
Introduction
While Google provides a robust ecosystem for Android development there are significant gaps where it relies on third-party libraries and companies to fill the void. From networking to database encryption developers often turn to external solutions for critical app functionality.
This article dives into five areas where Google’s solutions fall short the third-party libraries that developers rely on and the companies behind these libraries.
Code examples are included to briefly illustrate the libraries’ usage so you can get familiar with them.
1. Networking
Google’s low-level networking APIs such as HttpURLConnection
offer basic functionalities but lack the advanced features required for modern application development. To address these needs developers have turned to third-party libraries like Retrofit and Ktor which provide more comprehensive solutions for RESTful and GraphQL APIs.
Retrofit
Retrofit developed by Square was introduced in 2013 to simplify HTTP communication in Android applications. It offers a type-safe HTTP client for Java and Android allowing developers to define REST API interfaces using annotations. Retrofit handles the creation of network requests and responses integrating seamlessly with JSON parsers like Gson or Moshi for serialization and deserialization.
Over the years Retrofit has become a staple in Android development due to its simplicity and efficiency. Its widespread adoption is evident from its GitHub repository which boasts over 40000 stars and numerous forks indicating a large and active user base. The library is maintained by Square a company renowned for its contributions to open-source projects in the Android ecosystem.
Code Example with Retrofit:
// Define the API service interface
interface ApiService {
@GET("users/{id}")
suspend fun getUser(@Path("id") id: Int): User
}
// Create the Retrofit instance
val retrofit = Retrofit.Builder()
.baseUrl("https://api.example.com")
.addConverterFactory(GsonConverterFactory.create())
.build()
// Create an implementation of the API service
val apiService = retrofit.create(ApiService::class.java)
// Example call to fetch a user
val user = apiService.getUser(1)
Ktor
Ktor developed by JetBrains — the creators of Kotlin — was first released in 2018. It is a framework for building asynchronous servers and clients in connected systems using the Kotlin programming language. Ktor leverages Kotlin’s coroutines for asynchronous programming providing a flexible and efficient way to handle network operations.
Designed with a modular architecture Ktor allows developers to include only the features they need making it lightweight and adaptable. It supports both server-side and client-side development enabling the creation of microservices web applications and HTTP clients. Ktor’s integration with Kotlin Multiplatform projects facilitates code sharing across different platforms including Android iOS and the web.
Since its inception Ktor has gained traction among developers seeking a Kotlin-centric approach to networking. Its GitHub repository has garnered over 10000 stars reflecting a growing community of users and contributors. JetBrains actively maintains Ktor ensuring regular updates and improvements aligned with the evolution of the Kotlin language.
Code Example with Ktor
import io.ktor.client.*
import io.ktor.client.engine.cio.*
import io.ktor.client.features.json.*
import io.ktor.client.features.json.serializer.*
import io.ktor.client.request.*
// Initialize the HTTP client
val client = HttpClient(CIO) {
install(JsonFeature) {
serializer = KotlinxSerializer()
}
}
// Suspend function to fetch a user by ID
suspend fun fetchUser(id: Int): User {
return client.get("https://api.example.com/users/$id")
}
Adoption and Usage
Both Retrofit and Ktor have seen significant adoption in the developer community. Retrofit’s ease of use and integration with existing Java and Android projects have made it a go-to choice for many developers. Ktor’s modern coroutine-based approach appeals to those leveraging Kotlin’s advanced features especially in projects requiring asynchronous communication.
While exact usage statistics are challenging to determine the popularity of these libraries is evident from their widespread use in open-source projects tutorials and industry applications. Their active communities and regular updates ensure they remain relevant and reliable choices for developers addressing networking needs beyond what Google’s native APIs offer.
About the Companies:
- Square: Maintains Retrofit and OkHttp focusing on developer tools and payment solutions.
- JetBrains: Created Ktor a Kotlin-native library alongside Kotlin and IntelliJ IDEA.
2. Image Loading and Caching
When it comes to handling images efficiently in Android apps Google provides basic tools such as ImageView
and BitmapFactory
. However these are not optimized for modern app requirements like caching efficient decoding or handling large image files seamlessly. Developers often turn to third-party libraries for robust solutions as Google's native tools do not address the complexities of real-world use cases.
Why This is a Problem
Efficient image loading involves more than just fetching and displaying an image. It requires:
- Disk and Memory Caching: To avoid redundant downloads and improve performance.
- Placeholder Handling: To show fallback or loading states during fetch operations.
- Error Handling: To gracefully handle failed image requests.
- Image Transformation: Cropping resizing and applying filters without impacting performance.
- Compatibility with Modern UI Systems: Integration with both the legacy View System and Jetpack Compose.
Google doesn’t provide a unified or modern solution that covers all these aspects. Instead they rely on third-party vendors to fill the gaps.
Code Examples
Glide (View System)
Glide from Bump Technologies is widely used for its powerful caching mechanisms and extensive options for transformations. It integrates seamlessly with the View System:
Glide.with(context)
.load("https://example.com/image.png")
.into(imageView)
- Pros: Excellent caching smooth scrolling and flexible image transformations.
- Cons: Not designed for Jetpack Compose requiring workarounds or bridges for integration.
Coil (Jetpack Compose)
Coil developed by Instacart is a Kotlin-first library tailored for modern Android development and Jetpack Compose:
Image(
painter = rememberImagePainter("https://example.com/image.png")
contentDescription = "Example Image"
)
- Pros: Built specifically for Jetpack Compose lightweight and fully written in Kotlin.
- Cons: Newer compared to Glide and occasionally less feature-rich.
Picasso (View System)
Picasso created by Square was one of the first libraries to solve image loading problems. However it has seen reduced adoption in favor of Glide and Coil:
Picasso.get()
.load("https://example.com/image.png")
.into(imageView)
- Pros: Simple API good for legacy projects.
- Cons: Limited features compared to Glide and Coil and no Jetpack Compose support.
View System vs. Jetpack Compose
One of the challenges developers face is the fragmentation between Android’s UI systems. Glide and Picasso were designed primarily for the View System while Coil was built with Jetpack Compose in mind.
- View System (ImageView): Requires libraries like Glide or Picasso to handle image loading effectively.
- Jetpack Compose (Image): Needs libraries like Coil which integrate seamlessly with composable functions but may lack the maturity and performance optimization of older libraries.
3. Dependency Injection
Dependency Injection (DI) is a key architectural pattern that helps manage dependencies and promotes testable scalable code. While Google provides Hilt a simplified wrapper around Dagger it is still far from being a one-size-fits-all solution. Developers often find themselves exploring alternative tools like Koin to meet specific project requirements.
Why This is a Problem
Effective DI requires:
- Scalable Architecture: Ensuring clean and manageable dependency graphs in both small and large projects.
- Ease of Use: Reducing boilerplate and making DI accessible to developers of all levels.
- Kotlin-First Approach: Simplified syntax and integration in modern Android development.
- Integration Across Frameworks: Seamless DI support for the legacy View System and Jetpack Compose.
While Hilt addresses some of these concerns it heavily relies on Dagger which is complex and has a steep learning curve. This leaves many developers searching for simpler more intuitive alternatives.
Code Examples
Hilt
Hilt is Google’s solution for DI wrapping Dagger in an easier-to-use API. Here’s an example of its usage:
@HiltViewModel
class MyViewModel @Inject constructor(private val repository: Repository) : ViewModel()
- Pros: Strong integration with Android Jetpack components (e.g. ViewModel WorkManager etc.) easy to scale for large projects.
- Cons: Dependency on Dagger requires a fair amount of boilerplate and not as intuitive for beginners.
Koin
Koin from Insert-Koin.io offers a lightweight Kotlin-first alternative to Hilt. It simplifies DI and is favored in projects where Dagger or Hilt feels overly complex:
val appModule = module {
single { Repository() }
viewModel { MyViewModel(get()) }
}
startKoin {
androidContext(this@MyApplication)
modules(appModule)
}
- Pros: Minimal setup easy to understand Kotlin-friendly syntax and no annotation processing overhead.
- Cons: Lacks the deep integration with Jetpack components that Hilt provides and may not scale as effectively in very large projects.
About the Companies
- Square (Dagger): Dagger remains the gold standard for DI in Java/Android projects and serves as the backbone for Hilt. Its complexity however often intimidates developers especially those new to DI.
- Insert-Koin.io: A community-driven project focused on simplicity and modern Android development. Koin is ideal for Kotlin-based projects especially those leveraging Jetpack Compose.
View System vs. Jetpack Compose
DI tools must support both the View System and Jetpack Compose to remain relevant.
- Hilt (View System and Jetpack Compose): Hilt provides annotations like
@HiltViewModel
making it easier to inject dependencies into Compose’sViewModel
. - Koin: While Koin works with both systems it shines in Compose due to its Kotlin-first approach and lack of annotation processing.
4. Encrypting the Database
Database encryption is critical for protecting sensitive user data especially in industries like finance healthcare or e-commerce. However Google’s Room ORM despite its popularity does not offer built-in encryption capabilities. Developers must rely on external solutions like SQLCipher or Realm to secure their databases effectively.
Why This is a Problem
Securing database storage involves:
- Key Management: Safely storing and retrieving encryption keys without exposing them to unauthorized access.
- Performance Optimization: Ensuring encryption doesn’t degrade database read/write speeds.
- Compliance: Meeting industry standards for data protection (e.g. GDPR HIPAA).
Google’s Room ORM simplifies database management but leaves encryption entirely in the hands of third-party tools requiring extra setup and dependency management. This creates fragmentation and additional effort for developers.
Code Examples
SQLCipher with Room
SQLCipher from Zetetic is an established solution for SQLite database encryption. Here’s how it integrates with Room:
val passphrase = SQLiteDatabase.getBytes("secure_password".toCharArray())
val factory = SupportFactory(passphrase)
val db = Room.databaseBuilder(context AppDatabase::class.java "encrypted.db")
.openHelperFactory(factory)
.build()
- Pros: Strong encryption for SQLite-based databases well-documented and widely used.
- Cons: Requires additional setup and dependency management making it less seamless than built-in solutions.
Realm
Realm now owned by MongoDB offers built-in encryption as part of its database engine:
val config = RealmConfiguration.Builder()
.name("secure.realm")
.encryptionKey(ByteArray(64) { it.toByte() }) // Replace with your key
.build()
val realm = Realm.getInstance(config)
- Pros: Simple API for encryption easy integration and optimized performance for mobile apps.
- Cons: Locks you into the Realm ecosystem which may not fit all projects.
About the Companies
- Zetetic (SQLCipher): Renowned for its focus on database security and encryption for SQLite.
- MongoDB (Realm): A leader in NoSQL databases now leveraging Realm for mobile-first database solutions.
5. Advanced Logging and Analytics
Logging and analytics are crucial for monitoring app performance debugging issues and understanding user behavior. While Google provides Logcat for development and Firebase Crashlytics for crash reporting these tools lack flexibility and customization especially for advanced logging needs in production apps.
Why This is a Problem
Advanced logging and analytics require:
- Structured Logging: Categorizing and tagging logs for better filtering.
- Cross-Platform Consistency: Logging solutions that work seamlessly across Android server-side systems and other platforms.
- Flexible Configuration: Ability to switch log levels and outputs dynamically.
- Performance Optimization: Ensuring logging doesn’t slow down the app or bloat log files.
Google’s tools fall short in these areas making developers turn to third-party libraries like Timber and SLF4J for production-grade solutions.
Code Examples
Timber
Timber created by Jake Wharton is a lightweight and developer-friendly logging library for Android:
class MyApp : Application() {
override fun onCreate() {
super.onCreate()
if (BuildConfig.DEBUG) {
Timber.plant(Timber.DebugTree())
}
}
}
fun exampleLog() {
Timber.d("This is a debug log")
}
- Pros: Simplifies logging supports tagging and integrates seamlessly with Android.
- Cons: Focused on Android less suitable for multi-platform logging needs.
SLF4J
The Simple Logging Facade for Java (SLF4J) often paired with Logback is widely used in server-side Java applications but also serves Android projects:
val logger = LoggerFactory.getLogger("MyLogger")
fun logExample() {
logger.info("This is an info log")
}
- Pros: Works across platforms integrates with various logging backends (e.g. Logback Log4j).
- Cons: Requires more setup compared to Timber and less intuitive for mobile developers.
About the Companies
- Jake Wharton (Timber): A prominent open-source contributor who has created several essential Android libraries.
- SLF4J/Logback: Popular in the Java ecosystem for server-side logging adapted for Android in advanced use cases.
Conclusion
Google’s Android ecosystem provides a strong foundation but intentionally leaves gaps in critical areas such as networking dependency injection image handling database encryption and logging. To fill these gaps developers rely heavily on third-party libraries like Retrofit Koin Coil SQLCipher and Timber.
These external tools developed by companies like Square JetBrains and MongoDB or individual contributors like Jake Wharton play a vital role in enabling developers to build secure performant and feature-rich applications. While this fosters innovation it also adds complexity requiring developers to carefully evaluate and integrate external solutions.
Ultimately while Google offers the foundation third-party tools are essential for creating modern Android applications. Understanding and leveraging these complementary libraries is key to successful app development.

Dobri Kostadinov
Android Consultant | Trainer
Email me | Follow me on LinkedIn | Follow me on Medium | Buy me a coffee